What is React.js?
React.js is a popular JavaScript library for building user interfaces, particularly for single-page applications. It allows developers to create large web applications that can update and render efficiently with dynamic data changes.
Developed by Facebook, React.js uses a component-based architecture, enabling developers to build encapsulated components that manage their own state and can be composed to create complex UIs.
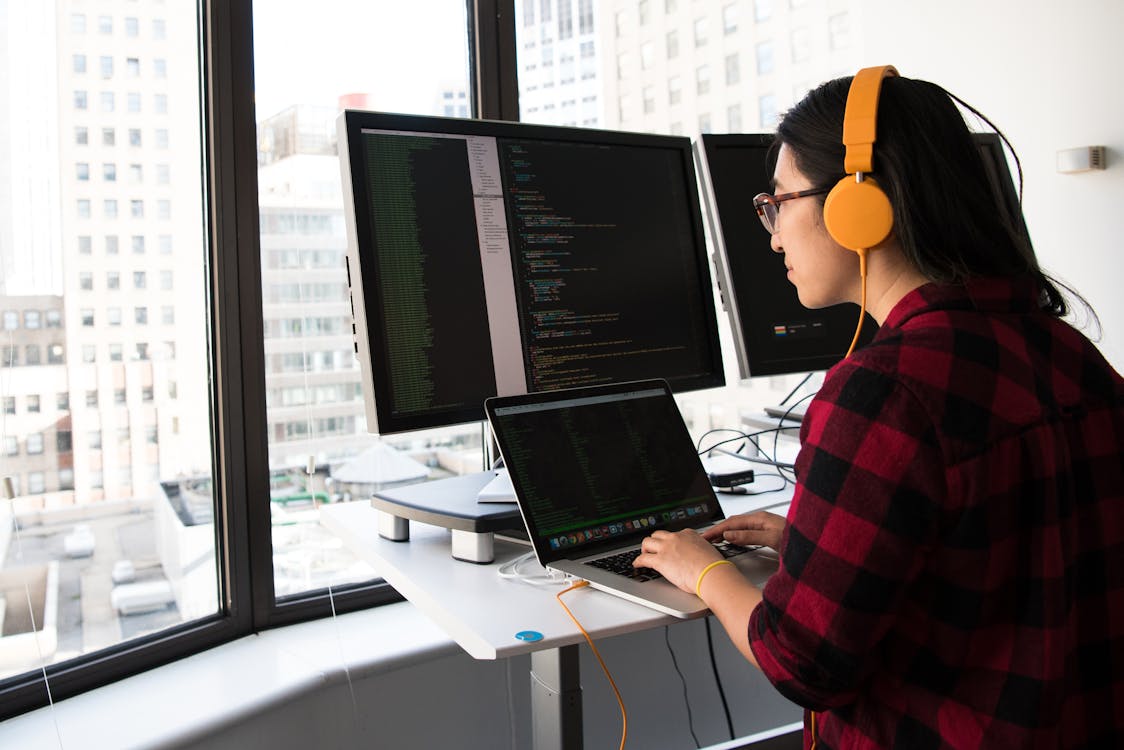
React.js Concepts
React.js is built around several core concepts that are crucial to understanding its capabilities:
- Components: The building blocks of a React application, components are self-contained units of code that represent parts of the UI.
- JSX: A syntax extension that allows mixing HTML with JavaScript, making it easier to write and understand the UI structure.
- State: An object that holds data and determines how a component renders and behaves.
- Props: Short for properties, these are inputs to components that allow data to be passed from one component to another.
- Virtual DOM: A lightweight copy of the actual DOM, allowing React to efficiently update and render components by minimizing direct manipulations.
React.js Features and Tools
React.js offers a variety of features and tools that enhance development and improve performance:
- Hooks: Functions that allow you to use state and other React features in functional components.
- Context API: A method to pass data through the component tree without having to pass props down manually at every level.
- React Router: A standard library for routing in React applications, enabling navigation between different views.
- Redux: A state management tool that helps manage application state in a predictable way.
- React DevTools: Browser extensions that help developers inspect and debug React applications more effectively.
Practical Tips for Working with React.js
To write efficient and maintainable React.js code, consider these best practices:
- Component Reusability: Build reusable components to reduce code duplication and enhance maintainability.
- Use Functional Components: Prefer functional components with Hooks over class components for simplicity and performance.
- Keep State Local: Avoid global state where possible to minimize complexity and improve performance.
- Leverage Prop Types: Use PropTypes to ensure your components receive the right data types and to catch errors early.
- Use Lazy Loading: Implement code-splitting and lazy loading to optimize the loading speed of your application.
Resources for Learning React.js
There are numerous resources available to help you master React.js:
- React Official Documentation: Comprehensive documentation straight from the source, covering all aspects of React.js.
- freeCodeCamp: Offers interactive tutorials and projects to help you build your React skills.
- Egghead.io: Short, expert-led video tutorials covering React.js topics in depth.
- Codecademy: Interactive courses that take you from the basics to advanced React.js concepts.
- W3Schools: Provides React.js tutorials, references, and examples to support your learning.
Conclusion
React.js is a powerful library that has transformed the way developers build web applications. By understanding its core concepts, leveraging its features, and following best practices, you can create dynamic, high-performance user interfaces. Continuous learning and practice are key to mastering React.js and contributing to the world of modern web development.