What is CSS?
CSS, or Cascading Style Sheets, is a stylesheet language used to describe the presentation of a document written in HTML or XML. CSS defines how elements should be rendered on screen, in print, or on other media. By separating the content from its visual styling, CSS enables greater flexibility and control over the layout, design, and overall look and feel of web pages.
CSS works hand-in-hand with HTML and JavaScript, where HTML structures the content, CSS styles it, and JavaScript adds interactivity. Understanding CSS is key to creating visually appealing and responsive web designs.
About CSS
CSS is composed of rulesets that target HTML elements and apply specific styles. A CSS ruleset consists of a selector and a declaration block.
Let's break down a basic CSS rule:
p {
color: blue;
font-size: 14px;
}
- Selector (p
): The selector targets the HTML element to be styled, in this case, all <p>
(paragraph) elements.
Declaration Block ({ ... }
): The declaration block contains the style rules to be applied. Each rule consists of a property (e.g., color
, font-size
) and a value (e.g., blue
, 14px
), separated by a colon.
Common CSS Properties
CSS properties define various aspects of styling, such as colors, fonts, and layout. Here are some commonly used CSS properties:
- color: Sets the color of the text. Example: `color: red;` will make the text red.
- background-color: Specifies the background color of an element. Example: `background-color: yellow;` sets a yellow background.
- font-family: Defines the font to be used for the text. Example: `font-family: Arial, sans-serif;` sets the font to Arial or a fallback sans-serif font.
- font-size: Sets the size of the font. Example: `font-size: 16px;` sets the font size to 16 pixels.
- margin: Controls the space outside an element. Example: `margin: 20px;` adds 20 pixels of space around the element.
- padding: Controls the space inside an element, between the content and its border. Example: `padding: 10px;` adds 10 pixels of space inside the element.
- border: Sets the border around an element. Example: `border: 1px solid black;` adds a 1-pixel solid black border.
- display: Specifies how an element is displayed on the page (e.g., `block`, `inline`, `flex`). Example: `display: flex;` makes the element a flex container.
- position: Controls the positioning of an element (e.g., `static`, `relative`, `absolute`, `fixed`). Example: `position: absolute;` positions the element relative to its nearest positioned ancestor.
- width/height: Sets the width and height of an element. Example: `width: 100px;` sets the width to 100 pixels.
- float: Allows an element to float to the left or right of its container. Example: `float: left;` makes the element float to the left.
- overflow: Specifies what happens if content overflows an element's box (e.g., `visible`, `hidden`, `scroll`). Example: `overflow: scroll;` adds scrollbars if the content overflows.
CSS Versions
CSS has evolved through various versions, each introducing new features and capabilities. Here's an overview:
- CSS1: Released in 1996, CSS1 introduced basic styling features such as fonts, colors, and text alignment.
- CSS2: Released in 1998, CSS2 added support for media types, positioning, and more sophisticated selectors.
- CSS2.1: A revision of CSS2, addressing issues and clarifying ambiguities.
- CSS3: Introduced in the late 2000s, CSS3 brought modularization, allowing individual features to evolve independently. It added support for transitions, animations, flexbox, grid layout, and more.
- CSS4: CSS4 is not an official version but a collection of CSS modules that are continuously evolving, introducing new features like custom properties (CSS variables) and advanced selectors.
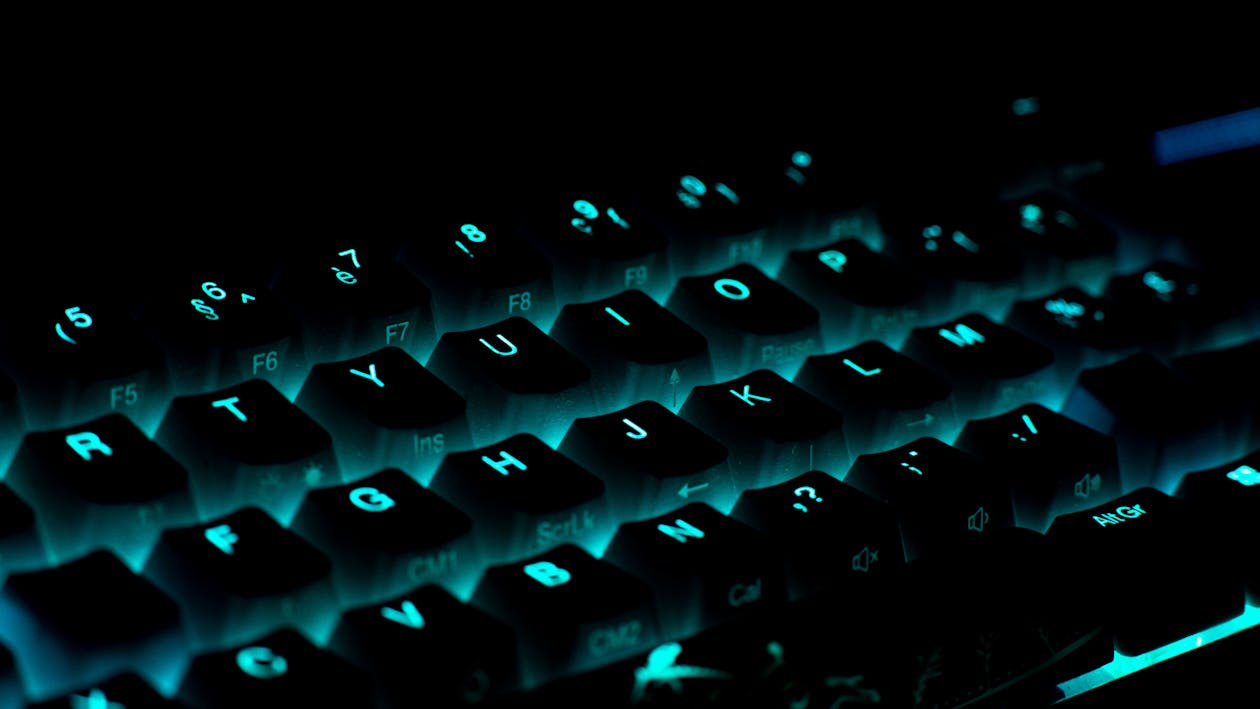
History of CSS
CSS was developed by Håkon Wium Lie and Bert Bos in 1996 to separate content from presentation. The early versions were relatively simple, but CSS has evolved to become a critical tool in web design and development.
With advancements in web technologies, CSS became more powerful, enabling developers to create complex layouts, animations, and responsive designs. Today, CSS is essential for building modern web interfaces.
Practical Tips for Working with CSS
To effectively work with CSS, consider these practical tips:
- Organize Your CSS: Group related styles and comment your code to make it easier to maintain and understand. Example:
/* Header Styles */
header {
background-color: #333;
color: white;
}
/* Footer Styles */
footer {
background-color: #f8f8f8;
color: #333;
} - Use a CSS Preprocessor: Tools like SASS or LESS allow you to use variables, nesting, and functions, making your CSS more manageable.
- Leverage Flexbox and Grid: Modern CSS layout techniques like Flexbox and Grid enable you to create complex layouts with ease.
- Keep It Responsive: Use media queries to ensure your design looks good on all devices. Example:
@media (max-width: 768px) {
body {
font-size: 14px;
}
} - Test Across Browsers: Ensure your CSS works consistently across different browsers and devices.
Conclusion
CSS is an indispensable tool for web developers. By mastering CSS, you can create visually appealing, responsive, and user-friendly websites that stand out. Whether you're a beginner or an experienced developer, there's always something new to learn in the world of CSS.