What is Angular?
Angular is a powerful, open-source web application framework developed and maintained by Google. It is designed to simplify the development and testing of single-page applications (SPAs) by providing a comprehensive set of tools and features. Angular uses TypeScript, a superset of JavaScript, and follows the MVC (Model-View-Controller) architecture.
Since its release in 2016, Angular has become one of the most popular frameworks for building dynamic, complex web applications. Its rich ecosystem, robust features, and active community make it a go-to choice for developers looking to create scalable and maintainable applications.
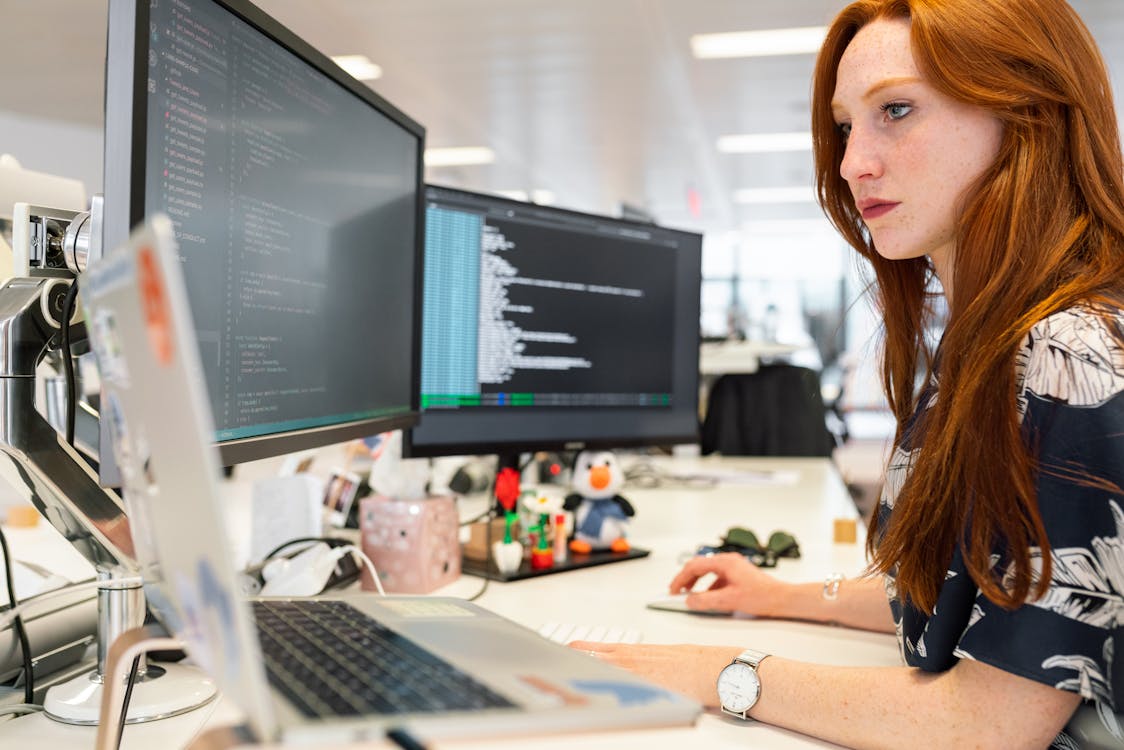
Angular Core Concepts
Angular is built around several core concepts that are essential to understand in order to use the framework effectively:
- Components: The building blocks of Angular applications, components encapsulate the logic, template, and styles for a specific part of the UI.
- Modules: Angular applications are organized into modules, which group related components, services, and other code into cohesive blocks.
- Services: Services provide a way to share data and logic across components. They are typically used for tasks like data fetching, state management, and business logic.
- Directives: Angular directives extend HTML by adding new behaviors to elements and components. Common directives include
*ngIf
and*ngFor
. - Routing: Angular's powerful routing module allows developers to define routes for different parts of their application, enabling navigation between views.
Advanced Angular Concepts
Angular offers a wealth of advanced features that enable developers to build sophisticated applications:
- Dependency Injection: Angular's dependency injection system allows services and other dependencies to be injected into components, making code more modular and testable.
- Change Detection: Angular's change detection mechanism keeps the UI in sync with the application's state. It efficiently tracks changes and updates the DOM only when necessary.
- Forms and Reactive Programming: Angular provides powerful tools for handling forms, including template-driven and reactive forms. Reactive forms, in particular, allow for more complex and scalable form management.
- State Management with NgRx: For larger applications, state management becomes crucial. NgRx is a popular library that integrates with Angular to manage application state using a reactive, store-based approach.
- Lazy Loading: Angular supports lazy loading, which allows parts of an application to be loaded on demand, reducing the initial load time and improving performance.
- Unit Testing: Angular has built-in support for unit testing with Jasmine and Karma, making it easier to write and run tests for components, services, and other parts of the application.
Angular Versions and Their Features
Angular has undergone significant evolution since its inception, with new versions bringing powerful features and improvements:
- Angular 2 (2016): A complete rewrite of AngularJS, introducing a component-based architecture, TypeScript, and improved performance.
- Angular 4 (2017): Introduced smaller and faster applications, with features like
ngIf
withelse
, and Angular Universal for server-side rendering. - Angular 6 (2018): Brought Angular Elements, the Angular CLI, and tree-shakable providers.
- Angular 9 (2020): Introduced the Ivy renderer, which improves build times and bundle sizes.
- Angular 11 (2020): Focused on improving developer experience, with features like stricter types, better error messages, and faster builds.
- Angular 14 (2022): Added typed forms, standalone components, and enhanced tooling support.
Best Practices for Angular Development
To write efficient and maintainable Angular applications, consider the following best practices:
- Follow the Angular Style Guide: Angular provides an official style guide that offers recommendations for writing clean and consistent code.
- Use TypeScript: TypeScript's strong typing and other features make it a perfect fit for Angular, helping to catch errors early and improve code quality.
- Optimize Performance: Use techniques like lazy loading, AOT compilation, and tree shaking to optimize the performance of your Angular applications.
- Write Modular Code: Break your application into modules and services to improve code organization and reusability.
- Keep Up with Angular Updates: Angular is continuously evolving, so staying updated with the latest versions and features is crucial for maintaining your applications.
- Test Thoroughly: Use Angular's built-in testing tools to write unit tests, end-to-end tests, and integration tests to ensure your application works as expected.
- Use Angular CLI: The Angular CLI simplifies many development tasks, from creating new components to running tests and optimizing builds.
Real-World Applications of Angular
Angular is used by many organizations to build complex and high-performance applications:
- Enterprise Applications: Angular's modular architecture and robust features make it ideal for building large-scale enterprise applications, such as customer relationship management (CRM) systems, enterprise resource planning (ERP) tools, and content management systems (CMS).
- Progressive Web Apps (PWAs): Angular's support for progressive web apps allows developers to create fast, reliable, and offline-capable applications that work on any device.
- eCommerce Platforms: Angular is a popular choice for building eCommerce platforms, thanks to its ability to handle dynamic content, complex user interactions, and seamless integration with payment gateways.
- Single-Page Applications (SPAs): Angular's powerful routing and state management capabilities make it perfect for building SPAs that provide a smooth and responsive user experience.
Practical Tips for Angular Developers
Here are some practical tips to help you get the most out of Angular:
- Understand the Angular Lifecycle: Familiarize yourself with Angular's component lifecycle hooks, such as
ngOnInit
andngOnDestroy
, to manage component state and side effects effectively. - Master RxJS: RxJS is an integral part of Angular's reactive programming model. Invest time in learning how to use observables, operators, and subjects to handle asynchronous data streams.
- Utilize Angular Material: Angular Material provides a set of UI components that follow the Material Design guidelines. It helps you create a consistent and visually appealing user interface quickly.
- Optimize for SEO: Angular applications can be optimized for search engines by using server-side rendering (SSR) with Angular Universal or pre-rendering techniques.
- Leverage Angular Schematics: Angular Schematics allows you to automate repetitive tasks, such as generating components, services, and modules, following best practices.
- Use Reactive Forms Wisely: Reactive forms provide a powerful way to manage form data and validation. Use them for complex forms that require dynamic behavior and validation logic.
- Participate in the Angular Community: The Angular community is vibrant and active. Engage with other developers through forums, social media, and conferences to stay updated and share knowledge.
Conclusion
Angular is a versatile and powerful framework that offers everything you need to build modern, high-performance web applications. Whether you're building small projects or large-scale enterprise applications, Angular provides the tools and features to help you succeed. By mastering Angular's core and advanced concepts, following best practices, and staying engaged with the community, you can become a proficient Angular developer and build applications that stand the test of time.
With continuous updates and a thriving ecosystem, Angular remains a top choice for developers looking to create cutting-edge web applications. So, dive into Angular, explore its features, and start building amazing applications today!