What is Node.js?
Node.js is a powerful, open-source, cross-platform runtime environment that allows developers to execute JavaScript code outside of a web browser. Built on Google Chrome's V8 JavaScript engine, Node.js enables server-side scripting, allowing developers to create scalable and efficient applications.
With Node.js, JavaScript has evolved from being just a client-side scripting language to a full-fledged server-side programming language. Its non-blocking, event-driven architecture makes it ideal for building real-time applications like chat servers, gaming platforms, and more.
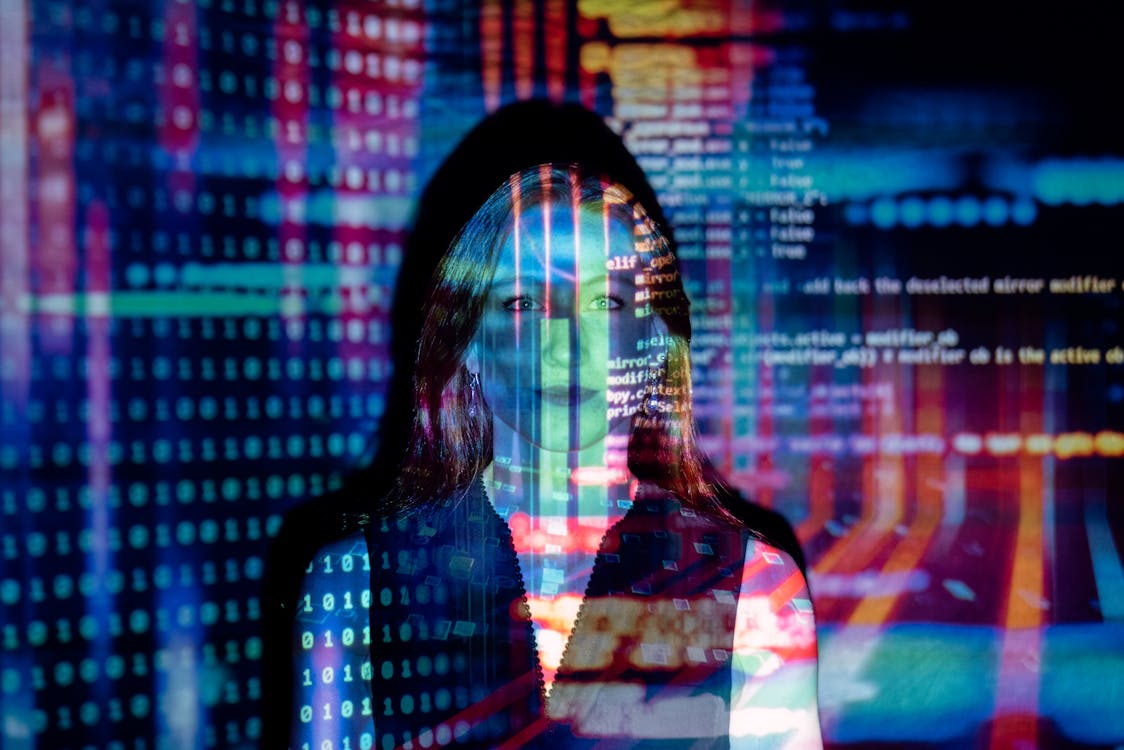
Core Concepts of Node.js
Node.js is built around several core concepts that are essential to understand in order to use the platform effectively:
- Event-Driven Architecture: Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications.
- Single-Threaded Event Loop: Despite being single-threaded, Node.js can handle multiple connections concurrently thanks to its event loop, which manages asynchronous operations.
- Asynchronous Programming: Node.js promotes asynchronous programming, where tasks can run in the background without blocking the main thread.
- Modules: Node.js has a rich set of built-in modules that provide functionalities like file I/O, networking, and more. Additionally, the Node Package Manager (NPM) allows developers to install and manage third-party modules.
Node.js Features and Their Benefits
Node.js offers a wide range of features that contribute to its popularity among developers:
- Fast Execution: Node.js uses the V8 engine, known for its high performance, allowing JavaScript code to be executed at a very high speed.
- Scalability: Node.js is highly scalable, thanks to its asynchronous nature, making it ideal for building scalable network applications.
- Rich Ecosystem: With NPM, Node.js has a vast ecosystem of libraries and packages, enabling developers to find solutions for almost any problem.
- Cross-Platform Compatibility: Node.js can run on multiple platforms including Windows, Linux, and macOS, making it a versatile choice for developers.
- Community Support: Node.js has a large and active community, offering a wealth of resources, tutorials, and support.
- Real-time Communication: Node.js is well-suited for building real-time applications like chat apps, collaborative tools, and live data streaming services.
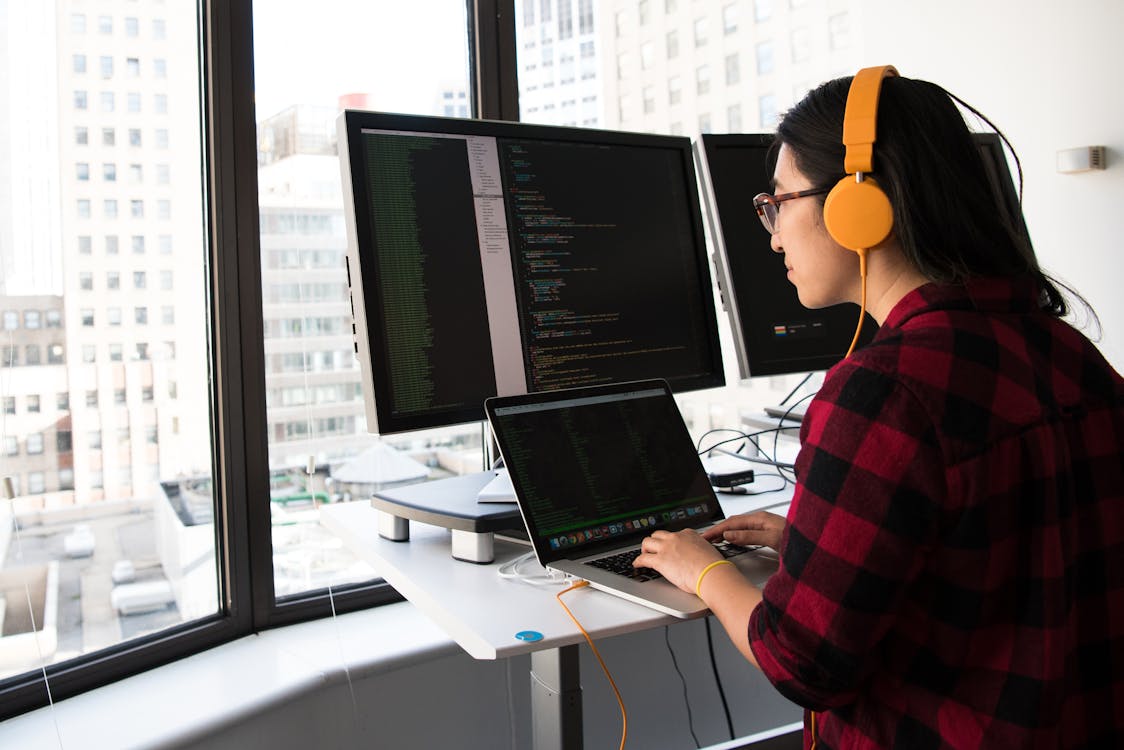
Advanced Node.js Features
Node.js is not only about basic features but also offers advanced capabilities that can enhance the development experience:
- Cluster Module: Enables the creation of child processes (workers) that share the same server port, allowing for the handling of more requests simultaneously.
- Stream API: Provides a powerful way to handle streaming data, such as reading or writing files, compressing data, and working with network requests.
- Worker Threads: Allows the creation of multiple threads within a Node.js process, facilitating multi-threaded execution in CPU-intensive tasks.
- Buffer: A raw binary data container, which can be used to handle binary data directly, especially in scenarios like file I/O.
Best Practices for Node.js Development
To make the most out of Node.js, it’s important to follow best practices that ensure the performance, security, and maintainability of your applications:
- Use Asynchronous Code: Embrace async/await patterns or promises to handle asynchronous operations effectively.
- Handle Errors Gracefully: Always include error handling in your code to prevent your application from crashing unexpectedly.
- Modularize Your Code: Break your code into smaller, reusable modules to enhance maintainability and scalability.
- Use Environment Variables: Store sensitive information like API keys and database credentials in environment variables instead of hardcoding them.
- Implement Security Best Practices: Protect your application from common security threats by validating input, using HTTPS, and keeping dependencies up to date.
Resources for Learning Node.js
There are plenty of resources available for developers who want to learn Node.js:
- Node.js Documentation: The official Node.js documentation provides a comprehensive guide to the platform, including tutorials and API references.
- freeCodeCamp: Offers an extensive curriculum that covers Node.js fundamentals and includes hands-on projects.
- Node School: A community-driven platform that provides interactive tutorials to learn Node.js in a practical manner.
- Udemy and Coursera: Provide a variety of courses ranging from beginner to advanced levels, allowing you to learn Node.js at your own pace.
- GitHub: Explore open-source Node.js projects on GitHub to understand how real-world applications are built.
Conclusion
Node.js has transformed the way JavaScript is used, enabling developers to create full-stack applications with a single language. Its event-driven, non-blocking I/O model, combined with a rich ecosystem of libraries, makes it a powerful tool for modern web development. By understanding its core concepts, leveraging its advanced features, and following best practices, you can build high-performance, scalable, and secure applications. Whether you are new to Node.js or looking to deepen your knowledge, continuous learning and hands-on experience are key to mastering this versatile runtime environment.