What is JavaScript?
JavaScript is a versatile, high-level programming language that enables developers to create dynamic and interactive web content. Initially developed to add interactivity to web pages, it has grown into a powerful tool that runs on both client and server sides, making it essential for modern web development.
JavaScript is often used alongside HTML and CSS to create a complete web experience. While HTML provides the structure and CSS handles the presentation, JavaScript adds the logic that powers interactive features like forms, animations, and real-time content updates.
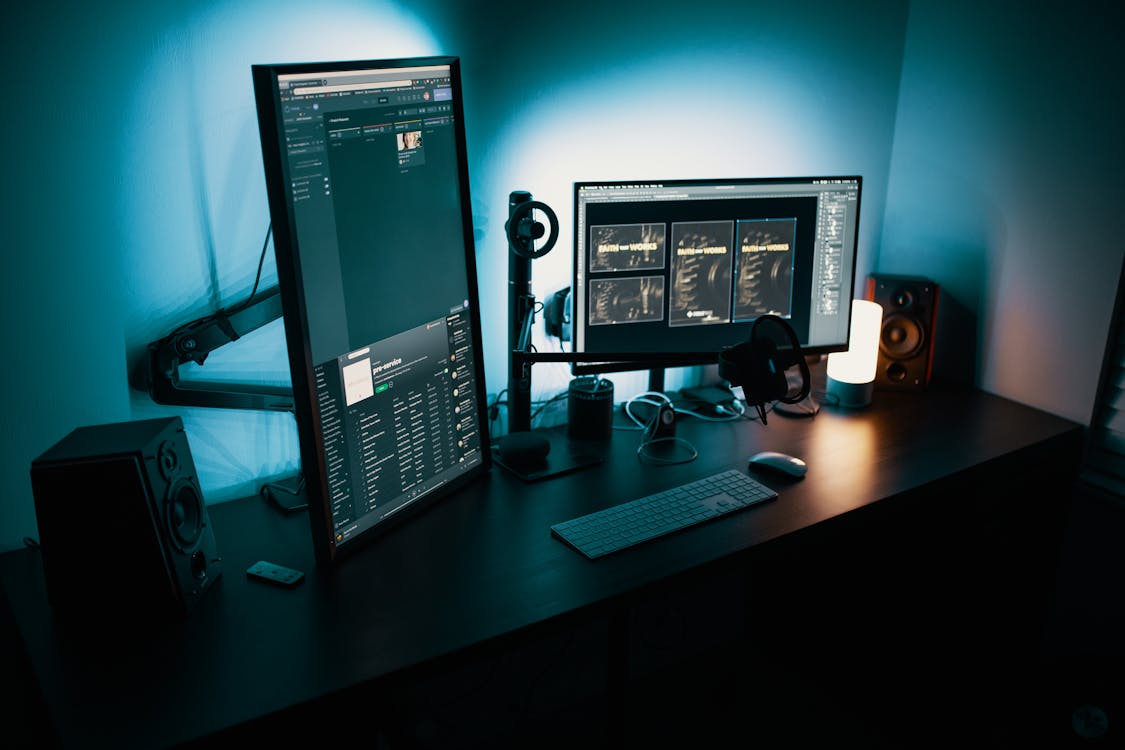
JavaScript Concepts
JavaScript is built around several core concepts that are essential to understand in order to use the language effectively:
- Variables: Containers for storing data values, declared using keywords like
var
,let
, orconst
. - Functions: Blocks of code designed to perform particular tasks. Functions can be declared traditionally or as arrow functions.
- Objects: Collections of properties and methods, used to model real-world entities and their behaviors.
- Arrays: Special types of objects used to store multiple values in a single variable.
- Control Flow: Structures like loops and conditionals that control the execution of code.
All JavaScript Topics and Their Details
JavaScript covers a broad range of topics that are crucial for both beginner and advanced developers:
- JS Iterables: Objects that can be iterated over, such as arrays and strings.
- JS Sets: Collections of unique values.
- JS Map Methods: Built-in methods for creating and managing key-value pairs.
- JS Classes: Templates for creating objects, allowing for object-oriented programming.
- JS Debugging: Tools and techniques for identifying and fixing errors in JavaScript code.
JavaScript Versions
JavaScript has evolved significantly since its inception, with new versions introducing powerful features and improvements. Here is a detailed overview of JavaScript versions:
- ES5 (2009): Introduced JSON support, new methods for arrays, and strict mode.
- ES6 (2015): A major update that included arrow functions, classes, modules, and more.
- ES2020: Added new features like optional chaining and BigInt.
- ES2021: Introduced logical assignment operators and other enhancements.
- ES2022: Brought features like top-level await and class fields.
Practical Tips for Working with JavaScript
To write efficient and effective JavaScript code, consider the following best practices:
- Use Strict Mode: Enforce stricter parsing and error handling in your JavaScript code by enabling strict mode.
- Optimize Performance: Use techniques like debouncing, throttling, and memoization to improve the performance of your JavaScript code.
- Stay Updated: Keep up with the latest ECMAScript versions to take advantage of new features and improvements.
- Write Modular Code: Break your code into reusable modules to improve maintainability and scalability.
- Use Modern Syntax: Embrace modern JavaScript syntax, such as arrow functions, destructuring, and template literals, to write cleaner, more concise code.
Resources for Learning JavaScript
There are numerous resources available to help you master JavaScript:
- Mozilla Developer Network (MDN): Offers comprehensive documentation and tutorials for all levels of JavaScript developers.
- JavaScript.info: A detailed online guide covering JavaScript fundamentals and advanced topics.
- freeCodeCamp: Provides an extensive JavaScript curriculum, including interactive challenges and projects.
- Codecademy: Offers interactive JavaScript courses for beginners and advanced learners alike.
- W3Schools: Features tutorials and references for JavaScript, including examples and exercises.
Conclusion
JavaScript is an essential technology in web development, powering the interactivity and logic behind modern web applications. By understanding its core concepts, keeping up with new versions, and following best practices, you can leverage JavaScript to create dynamic and engaging web experiences. Whether you’re a novice or an experienced developer, continuous learning and practice will help you excel in JavaScript development and contribute to the ever-evolving world of web technologies.