What is Python?
Python is a powerful, high-level programming language known for its readability and simplicity. It is widely used for web development, data analysis, artificial intelligence, scientific computing, and more. Python’s straightforward syntax makes it an ideal language for both beginners and experienced developers.
Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Its extensive standard library and vast ecosystem of third-party packages enable developers to tackle a wide range of projects with ease.
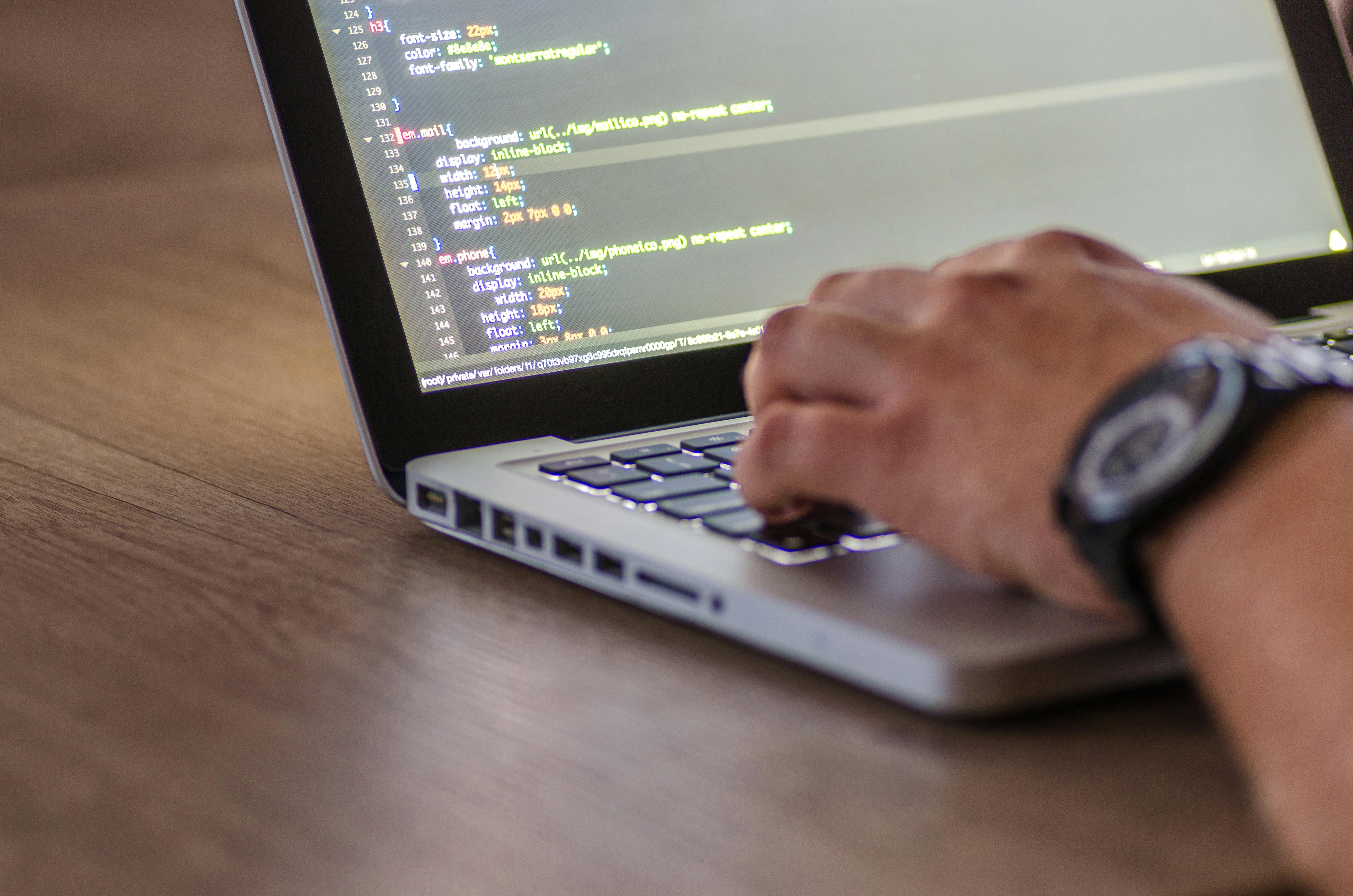
Python Concepts
Understanding core Python concepts is essential for effective programming:
- Variables: Python uses dynamic typing, meaning variables can change types as needed.
- Functions: Functions are defined using the
def
keyword and can return values using thereturn
statement. - Data Structures: Python has powerful built-in data structures like lists, dictionaries, and sets.
- Control Flow: Python supports if-else statements, loops, and exception handling to control the flow of programs.
- Object-Oriented Programming: Python allows for creating classes and objects, supporting encapsulation, inheritance, and polymorphism.
Advanced Python Features
Python offers several advanced features that can enhance your programming capabilities:
- Generators: Functions that return an iterator, allowing you to iterate over data without storing it in memory.
- Decorators: Functions that modify the behavior of other functions or methods, often used for logging or access control.
- List Comprehensions: A concise way to create lists by iterating over an iterable and optionally including conditions.
- Lambda Functions: Anonymous functions defined using the
lambda
keyword, often used for short, throwaway functions. - Context Managers: Simplify resource management using the
with
statement, handling setup and cleanup automatically.
Python Ecosystem
Python's ecosystem is vast, offering tools and libraries for almost every domain:
- Web Development: Frameworks like Django and Flask streamline web application development.
- Data Science: Libraries like NumPy, Pandas, and Matplotlib make data analysis and visualization accessible.
- Machine Learning: TensorFlow and PyTorch are popular frameworks for building machine learning models.
- Automation: Python can automate repetitive tasks with scripts, enhancing productivity.
- Testing: PyTest and Unittest are powerful tools for testing Python code, ensuring reliability.
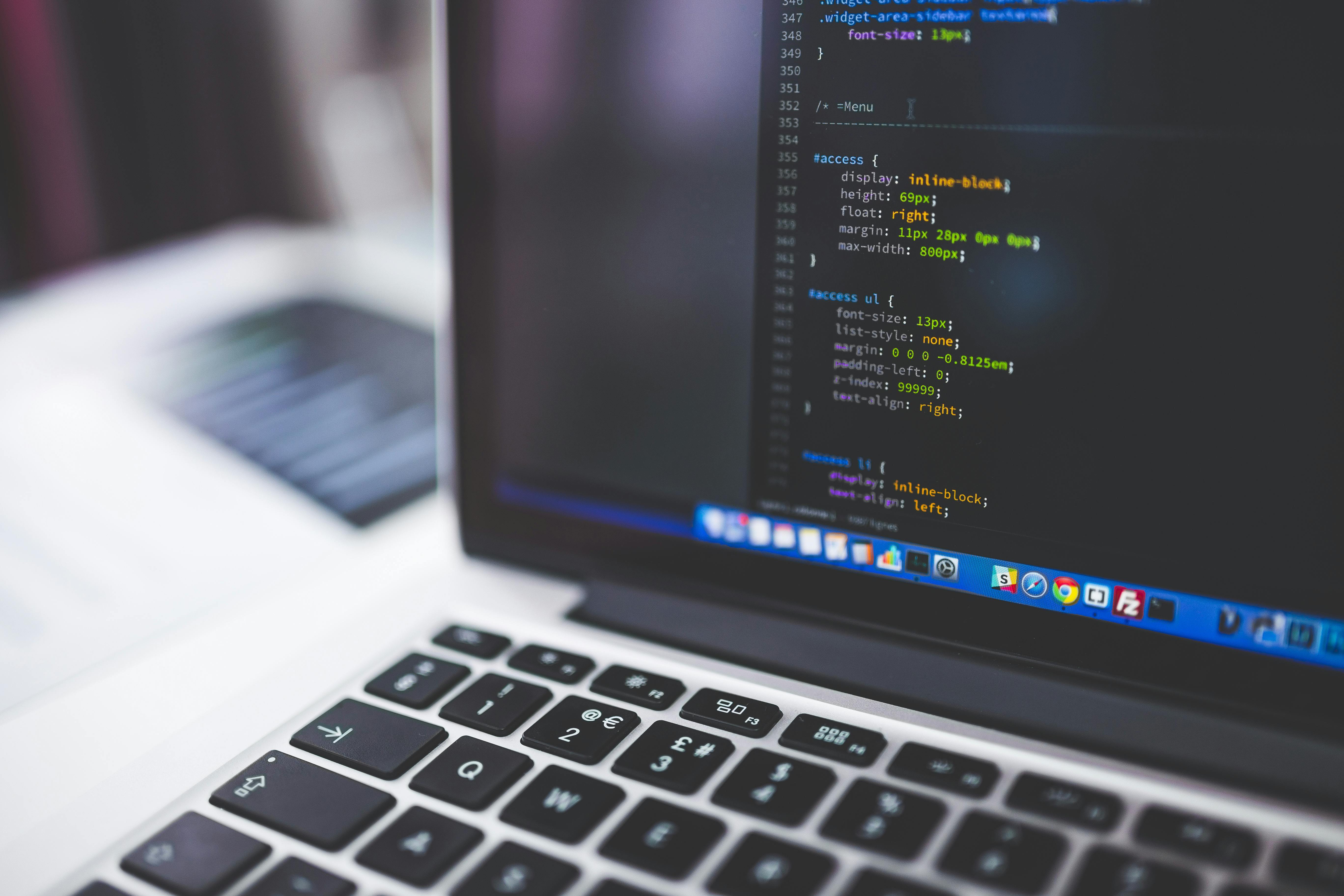
Best Practices for Python Development
Adopting best practices is key to writing clean, efficient, and maintainable Python code:
- Follow PEP 8: Python’s style guide, PEP 8, promotes consistency and readability in code.
- Use Virtual Environments: Isolate project dependencies using virtual environments to avoid conflicts.
- Write Tests: Ensure your code is reliable and bug-free by writing and running unit tests.
- Document Your Code: Use docstrings and comments to explain complex logic and functions.
- Leverage Libraries: Utilize Python’s rich ecosystem of libraries instead of reinventing the wheel.
Practical Python Tips
Here are some practical tips to help you excel in Python development:
- Optimize Performance: Use profiling tools to identify bottlenecks and optimize your code for speed.
- Keep Code DRY: Avoid duplication by following the "Don't Repeat Yourself" principle.
- Use Type Hinting: Python 3 supports type hints, which can improve code readability and reduce bugs.
- Master Debugging: Learn to use Python’s built-in debugger, pdb, to troubleshoot issues effectively.
- Stay Updated: Regularly update your Python knowledge by following Python Enhancement Proposals (PEPs) and participating in the community.
Resources for Learning Python
To further your Python skills, explore these learning resources:
- Python.org: The official Python website offers extensive documentation, tutorials, and guides.
- Real Python: A platform with articles, courses, and tutorials to help you become proficient in Python.
- Coursera: Offers courses on Python programming, data science, and machine learning.
- edX: Provides Python courses from top universities and institutions.
- Automate the Boring Stuff with Python: A practical book for learning Python by automating everyday tasks.
Conclusion
Python is a versatile and powerful programming language that can be used for a wide range of applications. By mastering its core concepts, taking advantage of its advanced features, and following best practices, you can become an effective Python developer. Continuous learning and practical application are the keys to success in Python development, and with the vast resources available, there's no better time to start or continue your Python journey.