What is Java?
Java is a versatile, high-level programming language that is widely used for building robust, scalable, and portable applications. It is known for its "write once, run anywhere" capability, allowing developers to run their Java code on any platform that supports the Java Virtual Machine (JVM).
Java is often used in enterprise environments, mobile applications, web development, and embedded systems. It is designed to be secure, fast, and reliable, making it a preferred choice for large-scale systems and critical applications.
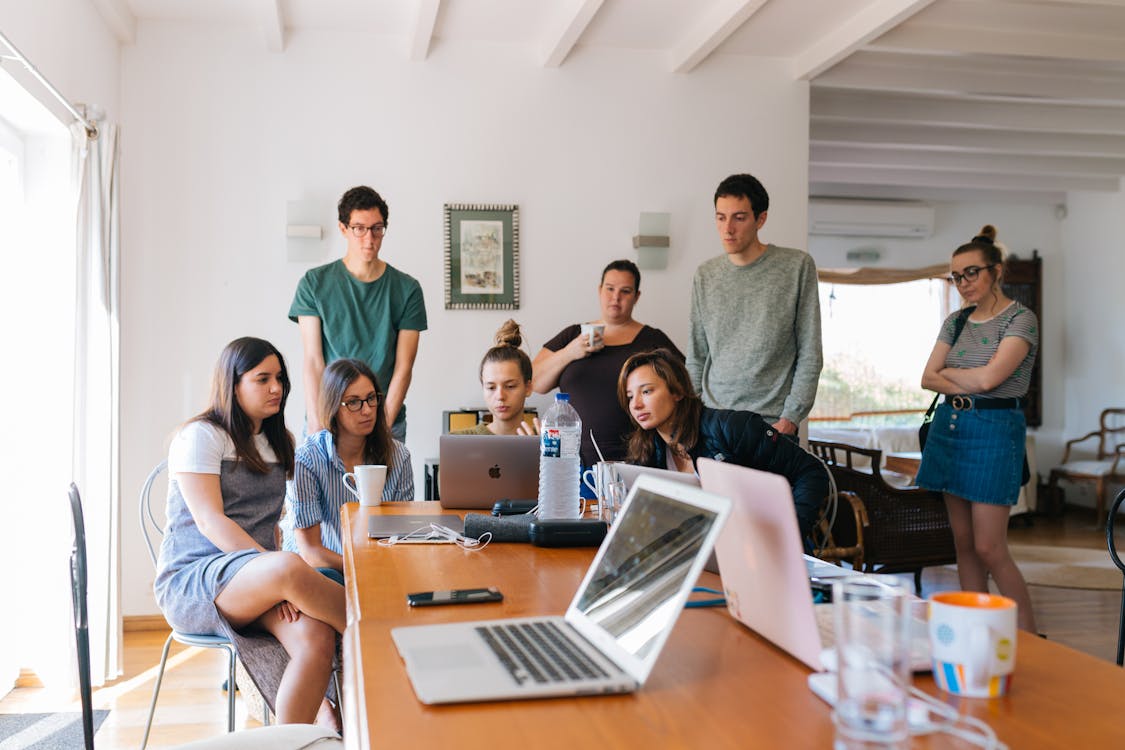
Java Core Concepts
Understanding Java requires a grasp of several fundamental concepts:
- Classes and Objects: Java is object-oriented, meaning it organizes software design around data, or objects, rather than functions and logic.
- Inheritance: Java allows new classes to inherit features from existing classes, promoting reusability and a hierarchical classification.
- Polymorphism: Java's ability to process objects differently based on their data type or class, making it flexible and extensible.
- Encapsulation: Bundling the data (variables) and the code (methods) that manipulates the data into a single unit called a class.
- Abstraction: Hiding the complex implementation details and showing only the essential features of an object.
Java Ecosystem
The Java ecosystem is vast, with tools and libraries that simplify development:
- Java Development Kit (JDK): The JDK provides the essential tools for developing Java applications, including the Java compiler, interpreter, and other tools.
- Java Runtime Environment (JRE): The JRE is a part of the JDK that enables running Java applications without the need for development tools.
- Integrated Development Environments (IDEs): Tools like IntelliJ IDEA, Eclipse, and NetBeans offer advanced features to enhance productivity.
- Frameworks: Popular Java frameworks like Spring and Hibernate streamline the development of enterprise and web applications.
- Libraries: Extensive libraries such as Apache Commons, Google Guava, and JUnit provide reusable code for common tasks.
Java Versions and Features
Java has seen numerous updates over the years, each introducing powerful features:
- Java SE 8: Introduced lambdas, the Stream API, and the java.time package for modern date and time API.
- Java SE 9: Brought the module system, which supports modular programming, and enhanced Javadoc.
- Java SE 11: Introduced the var keyword for local variable type inference and removed some deprecated features.
- Java SE 17: A long-term support release that included enhancements like sealed classes, pattern matching for switch, and records.
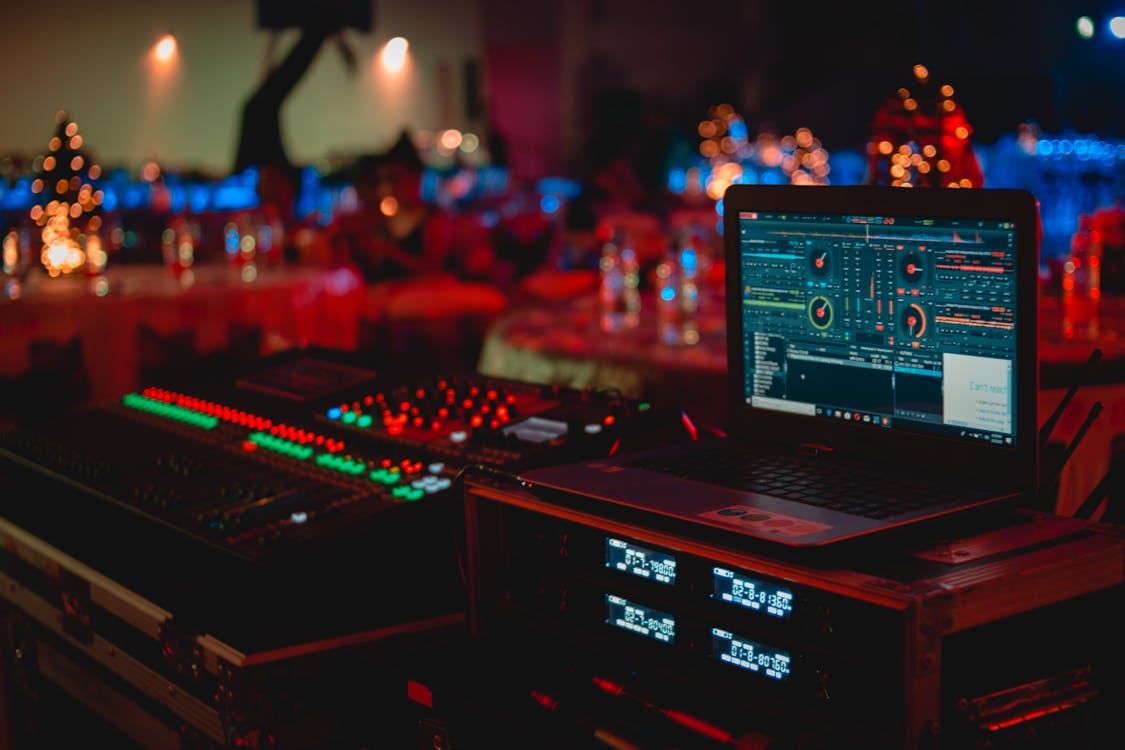
Advanced Java Topics
For developers looking to deepen their Java knowledge, exploring advanced topics is crucial:
- Concurrency: Learn about threads, synchronization, and the java.util.concurrent package for building multithreaded applications.
- Memory Management: Understanding Java's garbage collection and memory management is key to optimizing performance.
- Design Patterns: Familiarize yourself with common design patterns such as Singleton, Factory, and Observer.
- Networking: Java provides powerful APIs for building networked applications using sockets, RMI, and HTTP clients.
- Security: Java's security features include encryption, digital signatures, and secure communication protocols.
Best Practices for Java Development
To excel in Java development, consider these best practices:
- Follow Coding Standards: Adhere to coding standards and conventions to write clean, readable, and maintainable code.
- Optimize Performance: Regularly profile and optimize your code for better performance, especially in memory management and I/O operations.
- Write Unit Tests: Ensure your code is reliable by writing comprehensive unit tests using frameworks like JUnit and TestNG.
- Use Version Control: Track changes to your codebase using Git, and collaborate efficiently with others.
- Keep Learning: Stay updated with the latest Java releases and best practices by following community blogs, attending webinars, and participating in coding challenges.
Resources for Learning Java
Whether you're a beginner or an experienced developer, these resources can help you master Java:
- Oracle's Official Java Documentation: The official source for Java's syntax, APIs, and best practices.
- GeeksforGeeks: A comprehensive platform for tutorials, coding challenges, and interview preparation.
- Coursera: Offers Java courses from renowned universities and instructors, covering both basic and advanced topics.
- Codecademy: Provides interactive courses for beginners, focusing on hands-on practice and project-based learning.
- Java Programming Masterclass (Udemy): A popular course that covers Java fundamentals and advanced topics, with over 200 hours of content.
Conclusion
Java remains a dominant force in the programming world, offering unmatched versatility, scalability, and reliability. By mastering its core concepts, exploring advanced topics, and following best practices, you can build robust applications that meet modern demands. Whether you're developing enterprise solutions, mobile apps, or embedded systems, Java provides the tools and ecosystem to succeed.
Keep learning, experimenting, and refining your skills to stay ahead in the ever-evolving world of Java development.