What is PHP?
PHP (Hypertext Preprocessor) is a widely-used open-source scripting language designed for web development. It is a server-side language embedded within HTML, enabling the creation of dynamic web pages and applications.
PHP powers a vast number of websites and web applications, including content management systems (CMS), e-commerce platforms, and social networks. Its flexibility, ease of use, and integration with databases like MySQL make it a foundational tool for web developers.
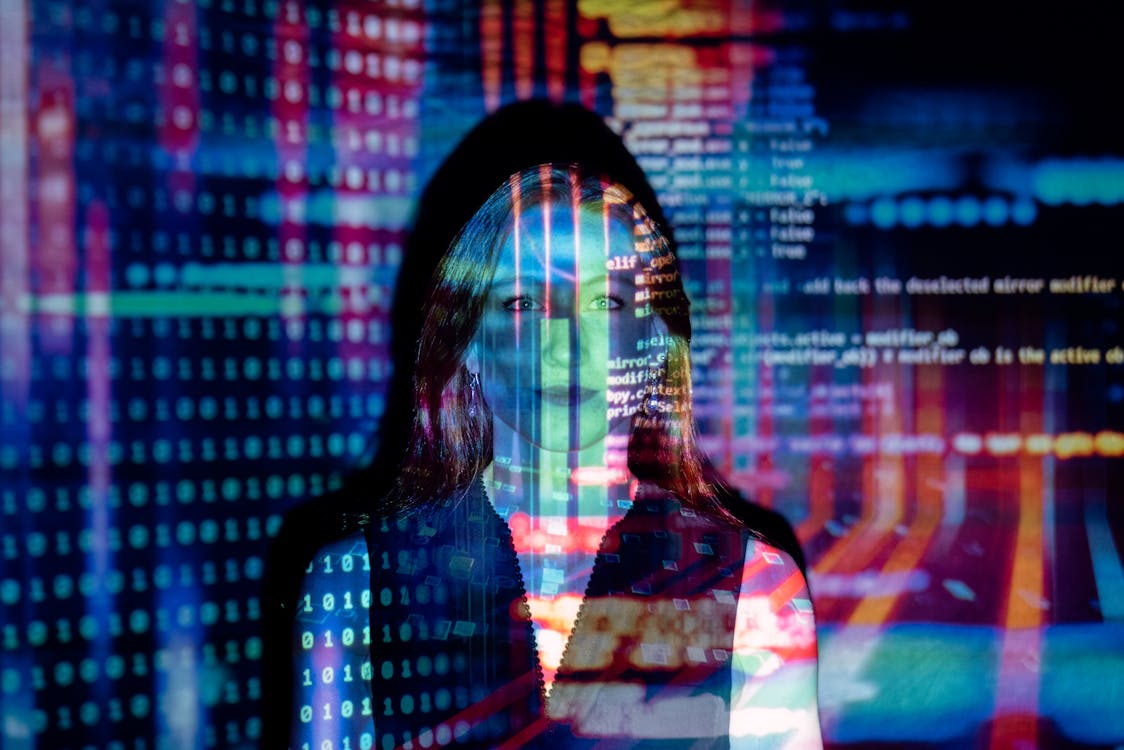
Core PHP Concepts
Understanding the fundamental concepts of PHP is crucial for effective web development:
- Variables: PHP variables are used to store data. They are declared with a
$
symbol followed by the variable name and can store different data types such as strings, integers, and arrays. - Functions: Functions are reusable blocks of code that perform specific tasks. PHP has a wide array of built-in functions and allows the creation of user-defined functions.
- Arrays: Arrays are collections of data items. PHP supports indexed arrays, associative arrays, and multidimensional arrays.
- Control Structures: PHP includes conditional statements and loops like
if
,else
,while
, andfor
, which control the flow of execution in a script. - Superglobals: PHP provides several superglobal variables such as
$_GET
,$_POST
, and$_SESSION
, which are available globally across scripts. - Data Types: PHP supports multiple data types, including strings, integers, floats, booleans, arrays, objects, and NULL.
Advanced PHP Features
PHP offers a range of advanced features that allow developers to build robust and scalable web applications:
- Object-Oriented Programming (OOP): PHP supports OOP principles, including classes, inheritance, and interfaces, allowing for more modular and maintainable code.
- Error Handling: PHP includes mechanisms such as
try...catch
blocks for handling exceptions and errors gracefully. - Session Management: PHP’s session management capabilities allow the storage of user data across multiple pages, essential for user authentication and personalized experiences.
- File Handling: PHP provides functions to read, write, and manipulate files on the server, which is useful for creating file-based applications.
- Security Features: PHP has built-in features for input sanitization, encryption, and protection against common security threats like SQL injection and XSS.
- Regular Expressions: PHP supports regular expressions for complex string manipulation, pattern matching, and data validation.
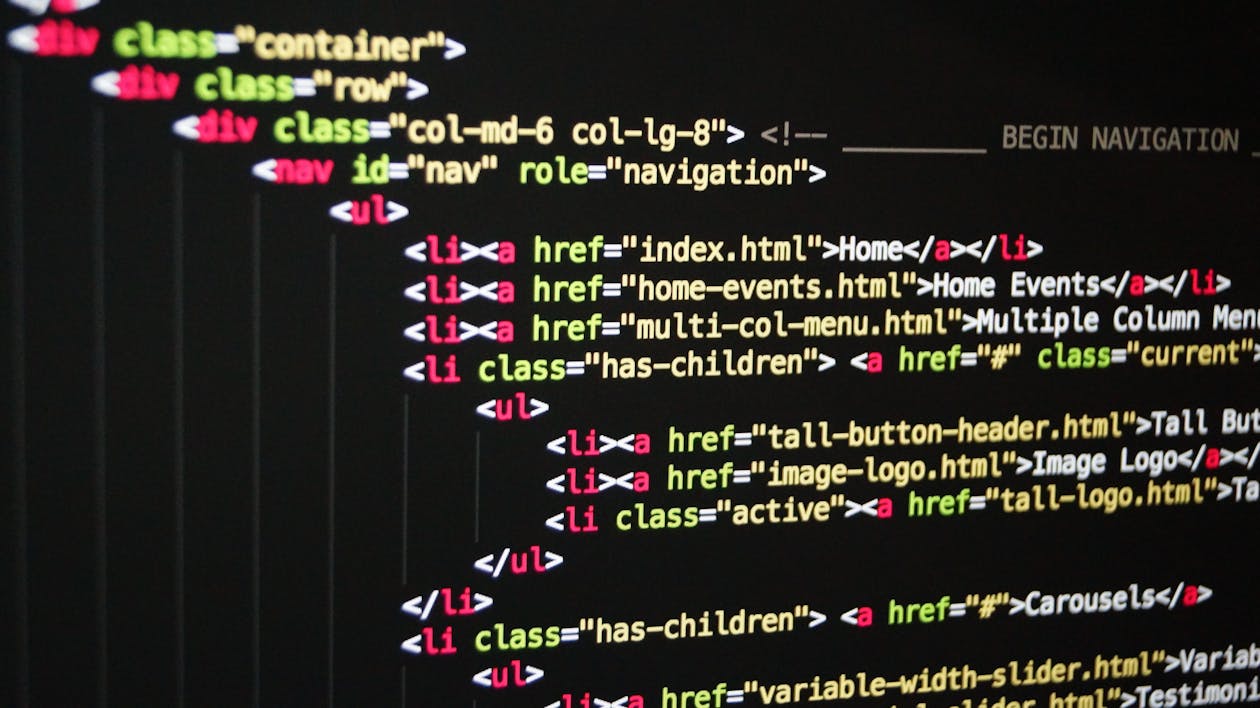
Database Integration with PHP
PHP is highly effective in interacting with databases, making it a preferred choice for building dynamic and data-driven applications:
- MySQL Integration: PHP’s built-in functions for MySQL enable easy database connection, querying, and management of data.
- PDO (PHP Data Objects): PDO is a database access layer that provides a consistent interface for interacting with multiple database types, offering better security and flexibility.
- ORM (Object-Relational Mapping): PHP frameworks like Laravel utilize ORM tools such as Eloquent to simplify database operations by mapping PHP objects to database records.
- MongoDB Integration: PHP can also integrate with NoSQL databases like MongoDB, offering flexibility in managing large datasets.
PHP Versions and Their Features
PHP has evolved significantly over the years, with each version introducing new features and improvements:
- PHP 5: Introduced significant features like OOP, exceptions, and improved MySQL support.
- PHP 7: Offered major performance enhancements, scalar type declarations, and error handling improvements.
- PHP 8: Brought Just-In-Time (JIT) compilation, union types, and further enhancements to error handling and the type system.
- PHP 8.1: Introduced enums, readonly properties, and performance improvements.
- PHP 8.2: Brought further refinements, such as readonly classes and type safety enhancements.
PHP Frameworks
PHP frameworks provide a structured approach to development, speeding up the process and enforcing best practices:
- Laravel: Laravel is a comprehensive MVC framework that offers features like routing, authentication, and database management out of the box.
- Symfony: Symfony is a highly flexible framework known for its reusable components and high performance, suitable for large-scale applications.
- CodeIgniter: CodeIgniter is a lightweight framework that is easy to set up and ideal for small to medium-sized projects.
- Yii: Yii is a high-performance framework designed for building modern web applications, offering features like caching and security measures.
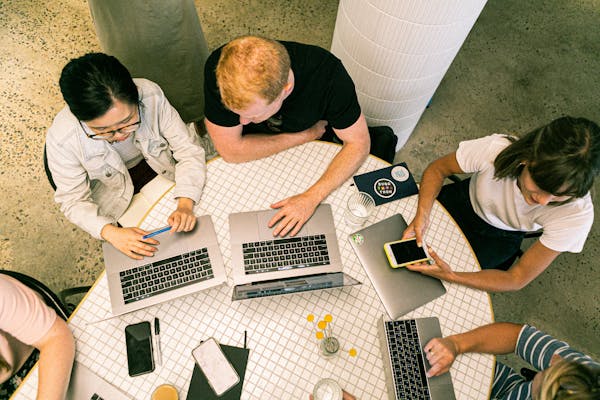
Practical Tips for Working with PHP
To enhance your PHP development skills, consider the following tips:
- Follow Coding Standards: Adhere to PHP coding standards such as PSR-12 to ensure code consistency and readability.
- Use Composer: Composer is a dependency management tool for PHP that simplifies the integration of third-party libraries into your projects.
- Leverage Testing: Implement unit testing with tools like PHPUnit to ensure your code functions as expected and is free of bugs.
- Optimize Performance: Optimize your PHP scripts by minimizing the use of loops, reducing database queries, and using caching mechanisms.
- Stay Updated: Keep up with the latest PHP updates and best practices by following the official PHP documentation and community forums.